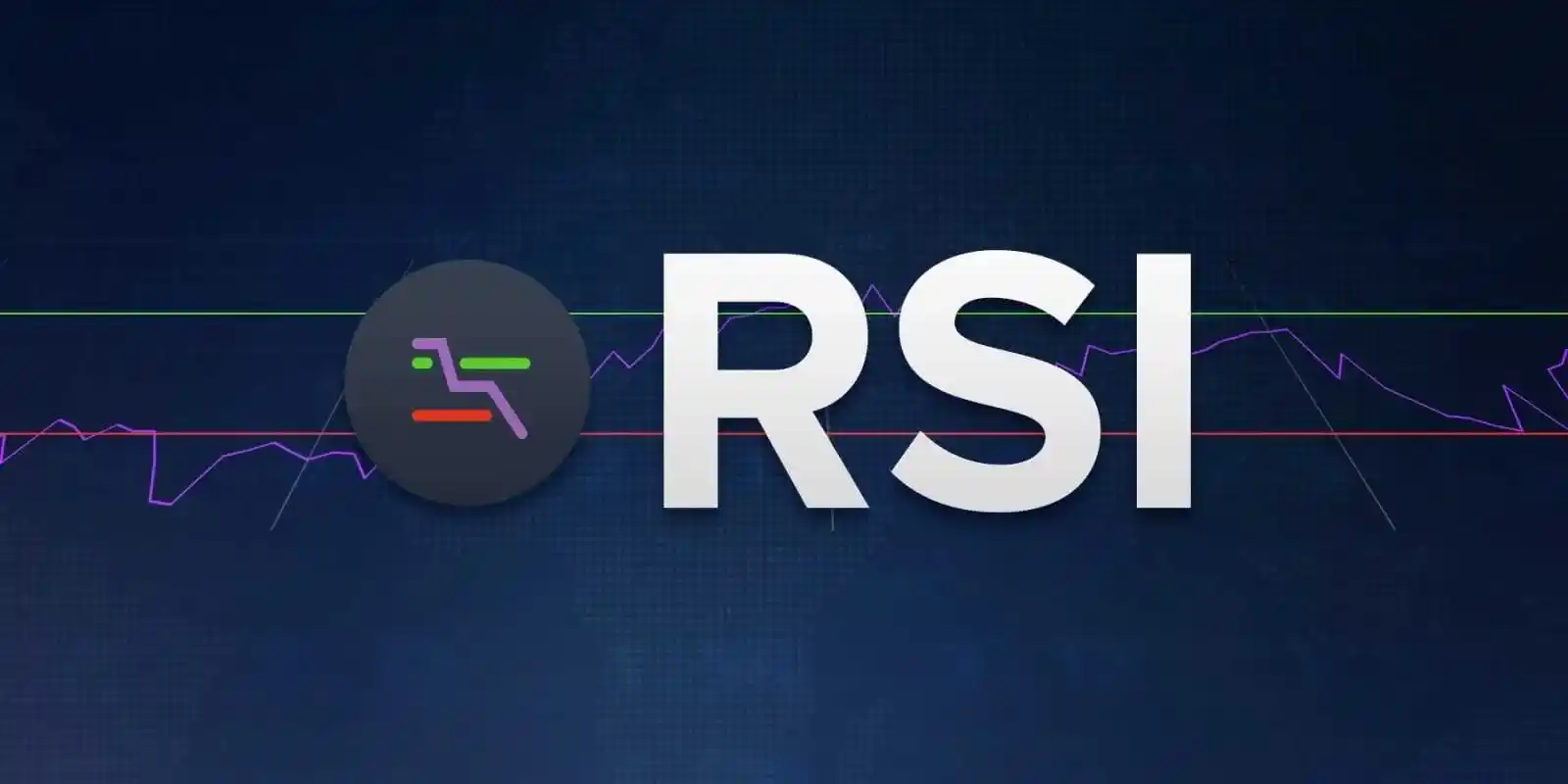
一、策略原理
相对强弱指标(Relative Strength Index, RSI)是由 Welles Wilder 提出的经典技术指标,通过计算价格波动的幅度衡量市场超买 / 超卖状态。RSI 取值范围 0-100,常用判断标准:
- RSI > 70:超买,潜在反转信号
- RSI < 30:超卖,潜在反转信号
- 结合趋势线或价格突破增强信号有效性
二、数据准备
本文使用 iTick 金融数据平台提供的高频数据,支持 A 股、期货、数字货币等多市场。安装数据接口库:
pip install itrade # iTick数据接口
数据获取示例(以沪深 300 指数期货为例):
""""
**iTick**:是一家数据代理机构,为金融科技公司和开发者提供可靠的数据源APIs,涵盖外汇API、股票API、加密货币API、指数API等,帮助构建创新的交易和分析工具,目前有免费的套餐可以使用基本可以满足个人量化开发者需求
https://github.com/itick-org
https://itick.org
""""
from itrade import quote
# 获取历史数据
df = quote.get_kline(
symbol="IF2303",
start_date="2023-01-01",
end_date="2024-01-01",
interval="1min"
)
# 数据预处理
df = df[['datetime', 'open', 'high', 'low', 'close', 'volume']]
df.set_index('datetime', inplace=True)
三、策略实现
1. RSI 计算函数
import pandas as pd
import numpy as np
def calculate_rsi(df, window=14):
delta = df['close'].diff(1)
gain = delta.where(delta > 0, 0)
loss = -delta.where(delta < 0, 0)
avg_gain = gain.rolling(window=window, min_periods=window).mean()
avg_loss = loss.rolling(window=window, min_periods=window).mean()
rs = avg_gain / avg_loss
rsi = 100 - (100 / (1 + rs))
df[f'RSI_{window}'] = rsi
return df
2. 信号生成逻辑
def generate_signals(df, rsi_window=14):
df = calculate_rsi(df, rsi_window)
# 金叉/死叉信号
df['signal'] = 0
df.loc[df[f'RSI_{rsi_window}'] > 70, 'signal'] = -1 # 超卖区做空
df.loc[df[f'RSI_{rsi_window}'] < 30, 'signal'] = 1 # 超买区做多
# 趋势过滤(可选)
df['ma50'] = df['close'].rolling(50).mean()
df.loc[df['ma50'] < df['ma50'].shift(1), 'signal'] = 0 # 下降趋势不做多
df.loc[df['ma50'] > df['ma50'].shift(1), 'signal'] = 0 # 上升趋势不做空
return df
四、策略回测
1. 基础回测框架
def backtest_strategy(df):
df['position'] = df['signal'].diff()
# 计算交易收益
df['returns'] = np.log(df['close'] / df['close'].shift(1))
df['strategy_returns'] = df['position'] * df['returns']
# 计算累计收益
df['cumulative_returns'] = df['strategy_returns'].cumsum()
# 计算年化收益、夏普比率等指标
total_days = len(df) / 252
sharpe_ratio = np.sqrt(252) * (df['strategy_returns'].mean() / df['strategy_returns'].std())
return df, sharpe_ratio
2. 回测结果分析
# 执行回测
df, sharpe = backtest_strategy(df)
print(f"策略夏普比率: {sharpe:.2f}")
print(f"最大回撤: {max_drawdown(df['cumulative_returns']):.2%}")
# 可视化
import matplotlib.pyplot as plt
plt.figure(figsize=(12,6))
plt.plot(df.index, df['cumulative_returns'], label='策略收益')
plt.plot(df.index, df['close'].pct_change().cumsum(), label='基准收益')
plt.legend()
plt.show()
五、策略优化方向
- 参数优化:使用 GridSearchCV 寻找最优 RSI 周期和阈值组合
- 多时间框架:结合日线与小时线信号提高胜率
- 风险控制:设置动态止损(如 ATR 通道止损)
- 资金管理:基于波动率调整仓位
六、iTick 数据优势
- 全市场覆盖:支持 A 股、期货、期权、数字货币等多品种
- 高频低延迟:提供 Level-2 行情和 Tick 级数据
- 便捷接入:支持 Python/R/Matlab 多语言接口
- 历史数据完整:提供十年以上历史行情数据
七、注意事项
- RSI 在趋势市场中可能出现钝化,建议结合趋势指标使用
- 需定期对策略参数进行再优化
- 实盘需考虑滑点和流动性风险
- 建议使用 iTick 的实时数据流进行策略验证
通过本文的代码框架,读者可以快速实现基于 RSI 的量化策略,并结合 iTick 的专业金融数据进行策略开发与验证。实际应用中需根据具体市场环境调整参数和风险控制规则。