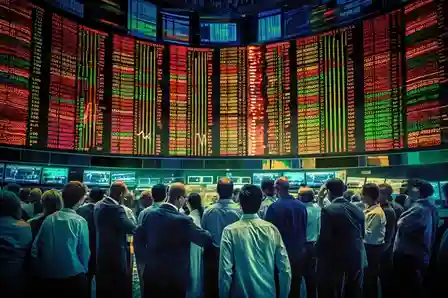
2025 实时高频外汇报价 API 对比
在金融市场浪潮中,实时高频外汇报价 API 如同投资者手中的指南针,精准的外汇高频报价数据更是左右投资决策的关键因素。对于企业、开发者以及投资者而言,如何在众多高频外汇 API 中挑选出契合自身需求的产品,已然成为在激烈市场竞争中抢占先机的核心要点。接下来,让我们一同深入剖析 iTick 平台在全球实时高频外汇报价 API 领域所展现出的显著优势。
市场主流外汇报价 API 现状
- 传统金融数据巨头:以彭博社为代表,在全球金融数据领域积淀深厚,数据权威全面。但外汇高频实时报价服务接入成本高,免费套餐功能有限、限制严格,中小开发者及预算有限企业难以承受。
- 综合性 API 平台:如 Rapids API,提供外汇实时与历史数据,支持双协议,传输灵活。不过免费版频率限制严格,付费成本高,无法满足高频数据需求的用户。
- 跨界数据方案:Google、Yahoo Finance 依赖第三方数据源,数据稳定性和时效性不确定。外汇交易时段数据延迟或中断,可能给专业用户造成经济损失。
iTick 平台的突出优势
- 平台服务质量:iTick 构建全球节点加速网络,能以极低延迟传输外汇数据,为高频交易策略提供保障。其专业运维团队 7*24 小时提供支持,随时解决用户技术问题,减少业务影响。
- 接口友好性:iTick 同时提供 RESTful API 和 WebSocket 实时推送通道,满足不同应用场景需求。基础免费版套餐无需繁琐手续,即可获取外汇指数行情和基础交易对数据,降低用户试错成本。
- 高频数据优势:iTick 的外汇 API 支持主要货币对毫秒级行情推送,提供 Bid/Ask 深度报价和实时波动率数据,帮助用户把握市场供需、调整交易策略。此外,平台还提供长达 15 年的日线级外汇历史数据,为量化交易和市场研究提供数据支撑。
实际应用案例
- 量化交易公司:某量化交易公司使用 iTick API 前,因数据延迟导致交易信号滞后,造成损失。切换后,利用毫秒级行情推送和稳定服务,优化交易策略,一个月内交易收益提升 30%,交易成本降低 20%。
- 金融科技初创企业:一家初创企业研发外汇交易分析工具时,面临数据接入难题。使用 iTick API 免费套餐完成原型开发,后升级付费套餐定制数据服务,开发出受欢迎的工具,半年内用户量增长 5 倍。
订阅示例
原始代码:https://github.com/itick-org/all-example/blob/main/nodejs/README.md
// 导入必要的模块
const WebSocket = require('ws'); // WebSocket客户端模块
const winston = require('winston'); // 日志记录模块
// 配置日志记录器
const logger = winston.createLogger({
level: 'info', // 设置日志级别为info
format: winston.format.combine(
// 添加时间戳,格式为:年-月-日 时:分:秒.毫秒
winston.format.timestamp({
format: 'YYYY-MM-DD HH:mm:ss.SSS'
}),
// 自定义日志输出格式
winston.format.printf(({ timestamp, level, message }) => {
return `${timestamp} ${level}: ${message}`;
})
),
// 配置日志输出目标:同时输出到控制台和文件
transports: [
new winston.transports.Console(), // 输出到控制台
new winston.transports.File({ filename: 'gold-price.log' }) // 输出到文件
]
});
/**
* 黄金价格订阅器类
* 用于连接WebSocket服务器,订阅黄金价格数据,并处理自动重连
*/
class GoldPriceSubscriber {
constructor() {
// WebSocket服务器地址
this.wsUrl = 'wss://api.itick.org/fws';
// 认证令牌
this.authToken = '***替换APIKey 请在 itick.io 官网申请***';
// 订阅的交易对符号
this.symbol = 'XAUUSD';
// WebSocket连接实例
this.ws = null;
// 连接状态标志
this.isConnected = false;
// 重连尝试次数
this.reconnectAttempts = 0;
// 最大重连尝试次数
this.maxReconnectAttempts = 100;
}
/**
* 建立WebSocket连接
* @returns {Promise} 返回连接成功或失败的Promise
*/
connect() {
return new Promise((resolve, reject) => {
try {
// 创建新的WebSocket连接
this.ws = new WebSocket(this.wsUrl);
// 监听连接打开事件
this.ws.on('open', () => {
logger.info('已成功连接到WebSocket服务器');
this.isConnected = true;
this.reconnectAttempts = 0; // 重置重连计数器
resolve(true);
});
// 监听数据接收事件
this.ws.on('message', (data) => {
// 使用上海时区记录接收时间
const timestamp = new Date().toLocaleString('zh-CN', { timeZone: 'Asia/Shanghai' });
logger.info(`[${timestamp}] 收到数据: ${data}`);
});
// 监听连接关闭事件
this.ws.on('close', async () => {
logger.warn('WebSocket连接已关闭');
this.isConnected = false;
await this.handleReconnection(); // 触发重连机制
});
// 监听错误事件
this.ws.on('error', (error) => {
logger.error(`WebSocket错误: ${error.message}`);
this.isConnected = false;
});
} catch (error) {
logger.error(`连接错误: ${error.message}`);
reject(error);
}
});
}
/**
* 发送认证信息
* @returns {Promise<boolean>} 返回认证是否成功
*/
async authenticate() {
if (!this.isConnected) {
logger.error('无法认证: 未连接到服务器');
return false;
}
try {
// 构建认证消息
const authMessage = {
ac: 'auth',
params: this.authToken
};
this.ws.send(JSON.stringify(authMessage));
logger.info('已发送认证请求');
return true;
} catch (error) {
logger.error(`认证错误: ${error.message}`);
return false;
}
}
/**
* 订阅行情数据
* @returns {Promise<boolean>} 返回订阅是否成功
*/
async subscribe() {
if (!this.isConnected) {
logger.error('无法订阅: 未连接到服务器');
return false;
}
try {
// 构建订阅消息
const subscribeMessage = {
ac: 'subscribe',
params: this.symbol,
types: 'tick'
};
this.ws.send(JSON.stringify(subscribeMessage));
logger.info(`已订阅 ${this.symbol} 行情数据`);
return true;
} catch (error) {
logger.error(`订阅错误: ${error.message}`);
return false;
}
}
/**
* 处理断线重连
* 包含延迟重试和最大重试次数限制
*/
async handleReconnection() {
if (this.reconnectAttempts >= this.maxReconnectAttempts) {
logger.error('已达到最大重连次数');
return;
}
this.reconnectAttempts++;
logger.info(`正在尝试重新连接 (第 ${this.reconnectAttempts} 次尝试)`);
try {
// 等待5秒后重连
await new Promise(resolve => setTimeout(resolve, 5000));
await this.start();
} catch (error) {
logger.error(`重连失败: ${error.message}`);
}
}
/**
* 启动订阅服务
* 按顺序执行:连接 -> 认证 -> 订阅
*/
async start() {
try {
await this.connect();
if (this.isConnected) {
await this.authenticate();
await this.subscribe();
}
} catch (error) {
logger.error(`启动失败: ${error.message}`);
await this.handleReconnection();
}
}
}
// 创建并启动订阅器实例
const subscriber = new GoldPriceSubscriber();
subscriber.start().catch(error => {
logger.error(`程序错误: ${error.message}`);
});