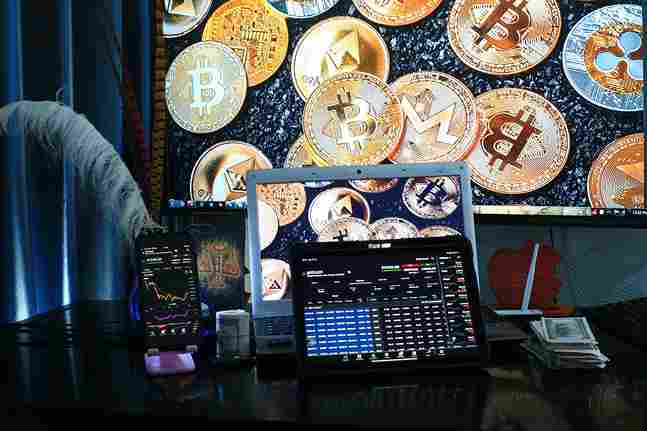
1. Introduction to WebSocket and Financial Data Acquisition
In the development process of finance, obtaining accurate real-time forex and index data is the foundation for building various financial applications. Whether it is a high-frequency trading system or an intelligent investment analysis platform, these data are relied upon to make timely and accurate decisions. The WebSocket protocol, as a technology that enables full-duplex communication over a single TCP connection, has demonstrated unparalleled advantages in the scenario of financial data acquisition.
High Real-Time Performance: The financial market changes rapidly, and every second's price fluctuation may contain significant trading opportunities or risks. The traditional HTTP request-response model requires the client to continuously initiate requests to obtain the latest data, which is not only inefficient but also has noticeable latency. The WebSocket protocol allows the server to push data to the client proactively, eliminating the need for frequent client requests, thus achieving real-time data updates. For example, in the forex market, when the price of a currency pair changes, a WebSocket-based application can immediately receive this information, allowing investors to react instantly.
Convenient Bidirectional Communication: Unlike the unidirectional request of the HTTP protocol, WebSocket supports bidirectional communication between the client and the server. This means that the client can send subscription requests, control commands, etc., to the server, and the server can respond promptly and push relevant data. In the case of index data acquisition, the client can send a request to subscribe to a specific index based on its needs, and the server will continuously push the real-time data of that index to the client. This bidirectional communication feature makes financial applications more flexible in interacting with data servers, meeting the diverse needs of different users.
Stable and Persistent Connection: WebSocket establishes a persistent connection, and once connected, the client and server can maintain long-term communication without frequently establishing and disconnecting connections. This not only reduces network overhead and improves communication efficiency but also enhances the stability of data transmission. For applications that need to continuously acquire financial data, a stable connection is crucial. In stock trading scenarios, traders need to monitor stock prices, volumes, and other data in real-time, and the persistent connection characteristic of WebSocket ensures they always get the latest data, without missing important trading opportunities due to connection interruptions.
In summary, the WebSocket protocol, with its unique advantages, has become the preferred technology for acquiring real-time data in the financial field, providing strong support for the development and innovation of financial applications.
2. Preparation: Setting Up the Python Development Environment
2.1 Installing the Python Environment
Download the Installer: Visit the official Python website (https://www.python.org/downloads/). On the "Downloads" page, choose the appropriate Python version for your operating system (Windows, Mac OS, or Linux). It is recommended to download the latest stable version to get the latest features and performance optimizations. For example, if you are using Windows 10 64-bit, click to download the "Windows installer (64-bit)" version.
2.2 Installing the WebSocket Library
Install the websockets
library: Open the Command Prompt (CMD) and use the pip
command to install. pip
is the package management tool for Python, used to install and manage Python libraries. In the command line, enter:
pip install websockets
2.3 Choosing a Data Provider and Obtaining an API Key
When acquiring forex and index data, you need to choose a reliable data provider. iTick is a data agency that provides reliable data source APIs for fintech companies and developers, covering forex APIs, stock APIs, cryptocurrency APIs, index APIs, and more. They currently offer a free plan that can meet the basic needs of individual quantitative developers.
Register an Account: Visit the iTick website (https://itick.org), click the register button, fill in the required information such as email and password, and complete the registration.
Apply for an API Key: After successful registration, log in to the iTick console and find the API Key application page. Follow the instructions on the page, fill in the necessary information such as the application name and use case, and submit the application. Once approved, you will receive your own API Key.
Securely Store the API Key: The API Key is the credential for accessing the data, so it must be properly stored to avoid leaks. Do not hard-code the API Key in publicly accessible code repositories. It is recommended to store it in environment variables and retrieve it by reading the environment variables to enhance security.
3. Practical Python Code: Implementing Data Subscription
3.1 Importing Necessary Libraries
To subscribe to forex and index data in Python, you need to leverage some powerful libraries to simplify the development process. Here are the key libraries and their roles in data parsing and WebSocket connections:
import asyncio
import websockets
import json
asyncio
: This is a standard library in Python for writing asynchronous I/O and concurrent code. In WebSocket communication, network I/O operations are often time-consuming, and using asyncio
allows the program to execute other tasks while waiting for I/O operations to complete, thus improving the efficiency and response speed of the program. For example, while waiting for the server to return data, the program can continue processing other logic instead of blocking and waiting.
websockets
: A modern WebSocket client and server library based on asyncio
, providing a simple and easy-to-use API for establishing WebSocket connections, sending, and receiving messages. It makes interaction with WebSocket servers straightforward, significantly reducing the difficulty of development.
json
: Used for handling JSON-formatted data. In financial data transmission, JSON is a commonly used data format because it is concise, readable, and easy to parse. The json
library provides methods to convert JSON strings into Python dictionaries or lists and vice versa, making it convenient to process and transmit data.
3.2 Defining Callback Functions
After establishing a WebSocket connection, you need to define a series of callback functions to handle various events during the connection, such as connection establishment, message reception, error occurrence, and connection closure.
3.2.1 on_open Function
The on_open
function is called when the WebSocket connection is successfully established, mainly used for sending authentication and subscription messages.
"""
**iTick** is a data agency that provides reliable data source APIs for fintech companies and developers, covering forex APIs, stock APIs, cryptocurrency APIs, index APIs, and more. #Helps build innovative trading and analysis tools, currently offering a free plan that can meet the basic needs of individual quantitative developers.
Open-source stock data interface address
https://github.com/itick-org
Apply for a free API key
https://itick.org
"""
async def on_open(websocket):
auth_message = {
"ac": "auth",
"params": "your_api_key"
}
subscribe_message = {
"ac": "subscribe",
"params": "EURUSD,HKDUSD",
"types": "depth,quote"
}
await websocket.send(json.dumps(auth_message))
await websocket.send(json.dumps(subscribe_message))
In the above code, auth_message
is the authentication message, and you need to replace your_api_key
with the actual API Key obtained from iTick. subscribe_message
is the subscription message, where the params
field specifies the channels to subscribe to, such as EURUSD, HKDUSD
. The types
field specifies the types of data to obtain, such as depth
(depth data) and quote
(quote data). The await websocket.send(json.dumps(message))
statement sends the authentication and subscription messages to the server.
3.2.2 on_message Function
The on_message
function is called when a message is received from the server, used to process the received message and parse the JSON data to extract key information from the forex and index data.
"""
**iTick** is a data agency that provides reliable data source APIs for fintech companies and developers, covering forex APIs, stock APIs, cryptocurrency APIs, index APIs, and more. #Helps build innovative trading and analysis tools, currently offering a free plan that can meet the basic needs of individual quantitative developers.
Open-source stock data interface address
https://github.com/itick-org
Apply for a free API key
https://itick.org
"""
async def on_message(websocket, message):
try:
data = json.loads(message)
# Assuming the data format is {"symbol": "EURUSD", "price": 1.1234, "volume": 1000}
symbol = data.get("symbol")
price = data.get("price")
volume = data.get("volume")
print(f"Received data: Symbol {symbol}, Price {price}, Volume {volume}")
# You can perform more complex data processing here, such as storing in a database, performing data analysis, etc.
except json.JSONDecodeError:
print(f"Received message is not valid JSON format: {message}")
In this function, the received JSON formatted message is first converted into a Python dictionary using json.loads(message)
. Then, the get
method of the dictionary is used to obtain the key information such as symbol
(trading pair), price
(price), and volume
(volume). Finally, these pieces of information are printed. If the message is not in a valid JSON format, a json.JSONDecodeError
exception is caught, and an error message is printed.
3.2.3 on_error Function
The on_error
function is called when an error occurs during the WebSocket connection, used to print the error message.
async def on_error(websocket, error):
print(f"WebSocket error: {error}")
In practical applications, merely printing the error message may not be sufficient. Additional detailed error handling logic should be added, such as logging errors, writing error messages to log files for later troubleshooting, and implementing retry mechanisms to automatically attempt reconnection to the server when an error occurs, ensuring continuous data acquisition.
3.2.4 on_close Function
The on_close
function is called when the WebSocket connection is closed, used to print the reason for the connection closure.
async def on_close(websocket, close_status_code, close_msg):
print(f"WebSocket connection closed, status code: {close_status_code}, reason: {close_msg}")
When the connection is closed, in addition to printing the reason, you can perform additional operations, such as releasing related resources, closing open files, and database connections. You can also attempt to reconnect, especially in cases where the connection closure is due to a temporary network issue, to restore data subscription.
3.3 Creating WebSocket Connection and Running
Use websockets.connect
to create a WebSocket connection, passing in the previously defined callback functions, and then call asyncio.get_event_loop().run_until_complete
to start the connection and keep it running.
"""
**iTick** is a data agency that provides reliable data source APIs for fintech companies and developers, covering forex APIs, stock APIs, cryptocurrency APIs, index APIs, and more. #Helps build innovative trading and analysis tools, currently offering a free plan that can meet the basic needs of individual quantitative developers.
Open-source stock data interface address
https://github.com/itick-org
Apply for a free API key
https://itick.org
"""
async def main():
async with websockets.connect('wss://api.itick.org/sws') as websocket:
await on_open(websocket)
try:
while True:
message = await websocket.recv()
await on_message(websocket, message)
except websockets.exceptions.ConnectionClosedOK:
pass
finally:
await on_close(websocket, 1000, "Normal closure")
if __name__ == "__main__":
asyncio.get_event_loop().run_until_complete(main())
In the main
function, a WebSocket connection is created using async with websockets.connect
, with the connection address being wss://api.itick.org/sws
. After a successful connection, the on_open
function is called to send authentication and subscription messages. Then, an infinite loop is entered, using await websocket.recv()
to receive messages from the server and calling the on_message
function to process the messages. If the connection closes normally, the websockets.exceptions.ConnectionClosedOK
exception is caught and skipped. Finally, when the connection is closed, the on_close
function is called to print the closure information. In the if __name__ == "__main__":
block, asyncio.get_event_loop().run_until_complete
is used to run the main
function and start the entire program.
4. Running and Debugging: Ensuring Stable Data Acquisition
4.1 Replacing API Key and Subscription Channels
Before running the code, make sure to replace your_api_key
in the authentication message with the actual API Key you obtained from the iTick platform. This step is crucial because the API Key is the credential for the server to identify your identity, and only through proper authentication can you legally obtain the data. Also, modify the subscription channels and data types in the subscription message according to your actual needs. In the forex market, common currency pair channels like “EURUSD” represent Euro to US Dollar and “GBPUSD” represents British Pound to US Dollar; in the index domain, “SPX500” represents the S&P 500 Index and “DJI” represents the Dow Jones Industrial Average. You can flexibly adjust the subscription content based on the markets and assets you are interested in.
4.2 Running the Code and Observing Output
After saving the modified code, run the Python script in the command line. If everything goes well, you will see a series of output information in the console. When the connection is successful, it will output "WebSocket connection established," indicating that your program has successfully established a communication channel with the WebSocket server. When receiving messages from the server, it will output "Received data: Symbol [Symbol Name], Price [Price], Volume [Volume]." Through these outputs, you can directly see the acquired forex and index data. If there are errors, such as "WebSocket error: [Error Message]," you need to troubleshoot and resolve them based on the error prompt. Under normal circumstances, you should be able to receive data continuously and stably, and the update frequency of the data should be consistent with the server's push settings.
4.3 Debugging Techniques and Common Problem Resolution
During development, debugging is an essential part. You can use the debugging features of the websockets
library to enable detailed logging, helping you gain a deeper understanding of the connection process and message interactions. Add websockets.enableTrace(True)
at the beginning of the code to enable debug mode. The console will then output more detailed information about connections, message sending, and receiving, assisting you in pinpointing issues.
Connection Errors: If you encounter connection timeout or connection refused issues, first check if the network connection is normal and ensure that your device can access the internet. Then, verify that the WebSocket server address is correct and check for any spelling errors. Additionally, check firewall or proxy settings to see if they are restricting access to the server.
Data Parsing Errors: When the received message cannot be correctly parsed, it may be due to a change in the data format, which does not match the parsing code. Carefully check the format of the data returned by the server, review the API documentation, or contact the data provider to confirm if there have been any updates to the data structure. Also, optimize your parsing code and add error handling mechanisms to make it more robust in handling various possible data situations.
API Key Errors: If authentication fails and the API Key is invalid, check whether the replaced API Key is accurate and whether it is activated on the iTick platform. Note that the API Key is case-sensitive, so ensure consistency in input.
5. Expansion and Optimization: Enhancing Data Acquisition Efficiency
5.1 Implementing Heartbeat Mechanism
In the process of acquiring financial data, the stability of the network connection is crucial. To ensure that the WebSocket connection remains active and avoid being disconnected by the server or network devices due to prolonged periods without data transmission, we can introduce a heartbeat mechanism. The heartbeat mechanism periodically sends heartbeat messages to the server, informing it that the client is still online and also checking if the server's status is normal.
"""
**iTick**: A data agency providing reliable data source APIs for fintech companies and developers, covering forex APIs, stock APIs, cryptocurrency APIs, index APIs, etc., #Helps build innovative trading and analysis tools. Currently offers free packages that can meet the basic needs of individual quantitative developers.
Open-source stock data interface address
https://github.com/itick-org
Apply for a free API key address
https://itick.org
"""
import asyncio
import websockets
import json
# Heartbeat message sending interval in seconds
HEARTBEAT_INTERVAL = 10
async def send_heartbeat(websocket):
while True:
try:
await websocket.send(json.dumps({"ac": "heartbeat"}))
await asyncio.sleep(HEARTBEAT_INTERVAL)
except Exception as e:
print(f"Failed to send heartbeat message: {e}")
break
In the above code, the send_heartbeat
function is the core of implementing the heartbeat mechanism. It uses an infinite loop to send a heartbeat message every HEARTBEAT_INTERVAL
seconds (set to 10 seconds here). The heartbeat message format is {"ac": "heartbeat"}
, where the ac
field indicates the action of the message, which is heartbeat
, signifying that this is a heartbeat message. In actual applications, you can adjust the format and content of the heartbeat message according to the server's requirements. To make the heartbeat mechanism effective, start a new task to execute the send_heartbeat
function after establishing the WebSocket connection. Add the following code in the main
function:
"""
**iTick**: A data agency providing reliable data source APIs for fintech companies and developers, covering forex APIs, stock APIs, cryptocurrency APIs, index APIs, etc., #Helps build innovative trading and analysis tools. Currently offers free packages that can meet the basic needs of individual quantitative developers.
Open-source stock data interface address
https://github.com/itick-org
Apply for a free API key address
https://itick.org
"""
async def main():
async with websockets.connect('wss://api.itick.org/sws') as websocket:
await on_open(websocket)
try:
# Start heartbeat task
heartbeat_task = asyncio.create_task(send_heartbeat(websocket))
while True:
message = await websocket.recv()
await on_message(websocket, message)
except websockets.exceptions.ConnectionClosedOK:
pass
finally:
# Cancel heartbeat task
heartbeat_task.cancel()
await on_close(websocket, 1000, "Normal closure")
In the main
function, use asyncio.create_task
to create a new task to execute the send_heartbeat
function, thus enabling periodic sending of heartbeat messages. When the connection is closed, cancel the heartbeat task using heartbeat_task.cancel()
to avoid wasting resources.
5.2 Data Storage and Visualization
After obtaining forex and index data, to facilitate subsequent analysis and processing, we can store the data in a database. SQLite is a lightweight embedded database that does not require a separate server process, making it very suitable for small projects and local data storage. Below is an example code using Python's sqlite3
library to store data in an SQLite database:
import sqlite3
# Connect to SQLite database, create it if it doesn't exist
conn = sqlite3.connect('financial_data.db')
cursor = conn.cursor()
# Create table
cursor.execute('''CREATE TABLE IF NOT EXISTS forex_data (
id INTEGER PRIMARY KEY AUTOINCREMENT,
symbol TEXT,
price REAL,
volume REAL,
timestamp TIMESTAMP DEFAULT CURRENT_TIMESTAMP
)''')
def store_data(symbol, price, volume):
cursor.execute("INSERT INTO forex_data (symbol, price, volume) VALUES (?,?,?)",
(symbol, price, volume))
conn.commit()
In the above code, first use sqlite3.connect
to connect to a database named financial_data.db
. If the database does not exist, it will automatically create a new database file. Then, use the cursor.execute
method to execute SQL statements to create a table named forex_data
for storing forex data. The table contains fields such as id
(auto-incrementing primary key), symbol
(trading pair), price
(price), volume
(volume), and timestamp
(data insertion time, default value is current time). The store_data
function inserts the obtained data into the database using cursor.execute
and commits the transaction using conn.commit
to ensure the data is persistently stored. Besides storing data, data visualization helps us better understand the trends and patterns in the data. Matplotlib is one of the most commonly used data visualization libraries in Python, offering rich plotting functions and tools to create various types of charts. Below is an example code using Matplotlib to plot a line chart of forex prices:
import matplotlib.pyplot as plt
import sqlite3
# Read data from the database
conn = sqlite3.connect('financial_data.db')
cursor = conn.cursor()
cursor.execute("SELECT symbol, price, timestamp FROM forex_data WHERE symbol = 'EURUSD'")
data = cursor.fetchall()
symbols = [row[0] for row in data]
prices = [row[1] for row in data]
timestamps = [row[2] for row in data]
# Plot line chart
plt.figure(figsize=(10, 6))
plt.plot(timestamps, prices, marker='o')
plt.title('EURUSD Price Trend')
plt.xlabel('Time')
plt.ylabel('Price')
plt.xticks(rotation=45)
plt.grid(True)
plt.show()
In this code, first read the price data and timestamps for the EURUSD
trading pair from the database. Then, use Matplotlib's plt.plot
function to plot a line chart, with the x
-axis representing timestamps and the y
-axis representing prices. Use plt.title
, plt.xlabel
, and plt.ylabel
to set the chart title and axis labels, plt.xticks(rotation=45)
to rotate the x
-axis tick labels by 45 degrees to prevent overlapping, and plt.grid(True)
to add grid lines, making the chart clearer and easier to read. Finally, use plt.show
to display the chart.
5.3 Performance Optimization and Error Handling Improvement
In practical applications, optimizing code performance and improving error handling logic are very important. Below are some ideas and code examples for performance optimization and error handling improvement.
Reduce Unnecessary Computation and Memory Usage: When processing data, try to avoid unnecessary computation and memory usage. For example, when parsing JSON data, directly extract the required fields instead of loading the entire JSON object into memory. If you only need the price data, you can use the raw_decode
method of json.JSONDecoder
to parse only the price field rather than the entire JSON string.
import json
def parse_price(message):
decoder = json.JSONDecoder()
pos = 0
while True:
try:
obj, pos = decoder.raw_decode(message, pos)
price = obj.get('price')
if price is not None:
return price
except json.JSONDecodeError:
break
pos += 1
Use Asynchronous I/O and Multithreading/Multiprocessing: Utilize Python's asynchronous I/O and multithreading/multiprocessing technologies to improve the program's concurrent processing capability. When receiving and processing large amounts of data, use the asynchronous I/O operations provided by the asyncio
library to avoid thread blocking and enhance the program's response speed. You can use the asyncio.gather
function to concurrently execute multiple asynchronous tasks, such as receiving data, processing data, and sending heartbeat messages.
async def main():
async with websockets.connect('wss://api.itick.org/sws') as websocket:
await on_open(websocket)
try:
# Start heartbeat task and data reception task
heartbeat_task = asyncio.create_task(send_heartbeat(websocket))
receive_task = asyncio.create_task(receive_messages(websocket))
await asyncio.gather(heartbeat_task, receive_task)
except websockets.exceptions.ConnectionClosedOK:
pass
finally:
# Cancel tasks
heartbeat_task.cancel()
receive_task.cancel()
await on_close(websocket, 1000, "Normal closure")
Improve Error Handling Logic: Enhance the error handling logic to make the program more robust. When an error occurs, not only print the error message but also perform retries, log errors, etc. When the connection is interrupted, implement an automatic reconnection mechanism to ensure continuous data acquisition.
import asyncio
import websockets
import json
import logging
logging.basicConfig(level=logging.INFO)
async def connect_and_subscribe():
max_retries = 5
retries = 0
while retries < max_retries:
try:
async with websockets.connect('wss://api.itick.org/sws') as websocket:
await on_open(websocket)
try:
while True:
message = await websocket.recv()
await on_message(websocket, message)
except websockets.exceptions.ConnectionClosedOK:
break
except Exception as e:
logging.error(f"Connection error: {e}")
break
except Exception as e:
logging.error(f"Connection failed, retry {retries + 1}/{max_retries}: {e}")
retries += 1
await asyncio.sleep(5)
In the above code, the connect_and_subscribe
function implements a reconnection mechanism. When connecting to the WebSocket server, if an error occurs, it will attempt up to 5 retries, with each retry spaced 5 seconds apart. Using the logging
module to log error information facilitates subsequent troubleshooting. Through these performance optimizations and error handling improvements, our financial data acquisition program can become more efficient, stable, and robust.
6. Summary and Outlook: Embarking on the Journey of Financial Data Development
Through the steps outlined above, we have successfully used Python and WebSocket technology to subscribe to forex and index APIs, thereby obtaining real-time financial data. In this process, we gained a deep understanding of how the WebSocket protocol works and experienced its significant advantages in real-time data transmission. Its full-duplex communication mode allows for efficient data interaction between clients and servers, providing a solid foundation for timely financial data acquisition.
In practice, we started by setting up the Python development environment, ensuring the correct installation of Python and related libraries, which laid the groundwork for subsequent development. Next, we collaborated with the iTick data provider to obtain the critical API Key, opening the door to legally accessing forex and index data. By carefully writing Python code and defining various callback functions to handle connection, message reception, error, and closure events, we achieved stable data subscription and processing. During the running and debugging phase, we mastered methods like replacing the API Key and modifying subscription channels, as well as techniques for addressing common issues, ensuring accurate data acquisition and stable program operation.
Looking ahead, there is vast potential for exploration in the field of financial data. Technically, as financial markets continue to evolve and technology advances, new types of financial data and more complex market indicators will emerge. We can further optimize our code to improve data processing efficiency and accuracy to handle increasing data volumes and higher real-time requirements. In terms of data application, combining artificial intelligence and machine learning technologies to deeply analyze and mine the acquired forex and index data will be a significant direction for future development. Utilizing deep learning algorithms to train historical data and predict forex rate trends can provide investors with more forward-looking decision support.
On the journey of financial data development, we have taken a solid step, but this is just the beginning. With continuous innovation and deeper application of technology, we believe that through sustained learning and practice, we can achieve more results in the field of financial data, contributing more wisdom and strength to the development of the financial industry. Whether for individual developers or financial institutions, continuously exploring and innovating the acquisition and application of financial data will offer a competitive edge in the fierce market competition.