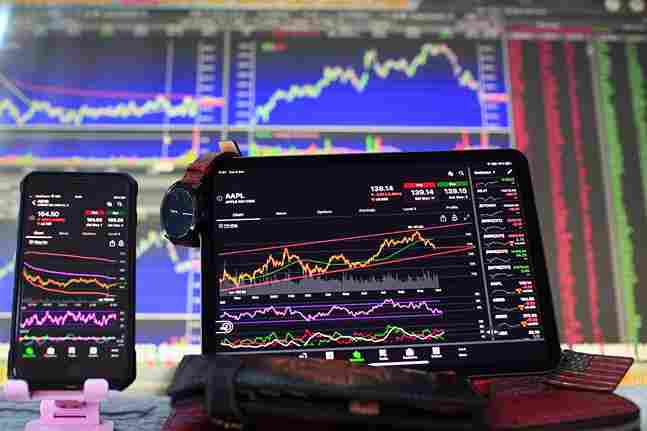
In financial development, obtaining real-time forex and index data is crucial. This tutorial will guide you on how to subscribe to free forex APIs and index APIs using the Java language, primarily through WebSocket technology to achieve real-time data acquisition.
Chapter One: Technical Principles
WebSocket is a protocol that enables full-duplex communication over a single TCP connection, allowing clients and servers to exchange data in real time. In this example, we use Java's WebSocket API to connect to the server providing forex and index data and subscribe to the required data channels.
Chapter Two: Preparation
Development Environment: Ensure you have installed the Java Development Kit (JDK) and configured the relevant environment variables.
IDE: It is recommended to use integrated development environments like IntelliJ IDEA or Eclipse for easier code writing and debugging.
API Service: This example uses wss://api.itick.org/sws
as the WebSocket server address. Ensure this service is available. Additionally, you need to obtain your own API Key for authentication.
Chapter Three: Code Explanation
(a) Import Necessary Packages
import javax.websocket.ClientEndpoint;
import javax.websocket.CloseReason;
import javax.websocket.ContainerProvider;
import javax.websocket.OnClose;
import javax.websocket.OnError;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.WebSocketContainer;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
These packages provide core functionalities for using WebSockets in Java, including defining client endpoints, managing connections, and handling messages.
(b) Define WebSocket Client Endpoint
@ClientEndpoint
public class WebSocketSubscriber {
The @ClientEndpoint
annotation marks this class as a WebSocket client endpoint, used for interacting with the WebSocket server.
(c) Configure Server Address and Message Format
// WebSocket server address
private static final String WEBSOCKET_SERVER_URL = "wss://api.itick.org/sws";
// Authentication message
private static final String AUTH_MESSAGE = "{" +
"ac":"auth" +
"params":"you_apikey" +
"}";
// Subscription message format, assuming subscription to a channel named "your_channel"
private static final String SUBSCRIBE_MESSAGE = "{" +
"ac":"subscribe" +
"params":"AM.LPL,AM.LPL" +
"types":"depth,quote" +
"}";
WEBSOCKET_SERVER_URL
defines the WebSocket server address to connect to. AUTH_MESSAGE
is the authentication message, where you need to replace your_apikey
with your own API Key. SUBSCRIBE_MESSAGE
is the subscription message, with the params
field specifying the channels to subscribe to and the types
field specifying the data types to retrieve.
(d) Main Method
public static void main(String[] args) {
try {
// Create WebSocket container
WebSocketContainer container = ContainerProvider.getContainer();
// Connect to WebSocket server and get session
Session session = container.connectToServer(WebSocketSubscriber.class, new URI(WEBSOCKET_SERVER_URL));
// Send authentication message
session.getBasicRemote().sendText(AUTH_MESSAGE);
// Send subscription message
session.getBasicRemote().sendText(SUBSCRIBE_MESSAGE);
} catch (URISyntaxException | IOException e) {
e.printStackTrace();
}
}
In the main
method, first create a WebSocket container, then use it to connect to the specified WebSocket server and obtain a session. Next, send the authentication and subscription messages via the session.
(e) Event Handling Methods
@OnOpen
public void onOpen(Session session) {
System.out.println("WebSocket connection opened");
}
@OnMessage
public void onMessage(Session session, String message) {
System.out.println("Received message: " + message);
// Further processing of the received message can be done here, such as parsing JSON data
}
@OnError
public void onError(Session session, Throwable error) {
System.out.println("WebSocket error: " + error.getMessage());
}
@OnClose
public void onClose(Session session, CloseReason closeReason) {
System.out.println("WebSocket connection closed, reason: " + closeReason.getReasonPhrase());
}
The method annotated with @OnOpen
is called when the WebSocket connection is successfully opened. The method annotated with @OnMessage
is called when a message from the server is received, where you can further process the received message, such as parsing JSON data. The method annotated with @OnError
is called when an error occurs, and the method annotated with @OnClose
is called when the WebSocket connection is closed.
Chapter Four: Running the Code
Copy the above code into your Java project.
Replace your_apikey
in AUTH_MESSAGE
with your own API Key.
Modify the subscription channels and data types in SUBSCRIBE_MESSAGE
according to your needs.
Run the main
method and observe the console output. You should see connection status, received messages, and any possible error information.
Chapter Five: Precautions
API Key Security: Ensure your API Key is not exposed to avoid security risks.
Data Format Parsing: Parse the received messages correctly based on the data format provided by the API to obtain useful forex and index data.
Network Stability: Since WebSocket relies on network connectivity, ensure network stability to avoid data loss or connection interruptions.
By following the above steps, you should be able to successfully subscribe to free forex APIs and index APIs using the Java language and obtain real-time financial data. We hope this tutorial is helpful to you!
iTick: A data agency providing reliable data source APIs for fintech companies and developers, covering forex APIs, stock APIs, cryptocurrency APIs, index APIs, etc., #Helps build innovative trading and analysis tools. Currently offers free packages that can meet the basic needs of individual quantitative developers.
Open-source stock data interface address
https://github.com/itick-org
Apply for a free API key address
https://itick.org