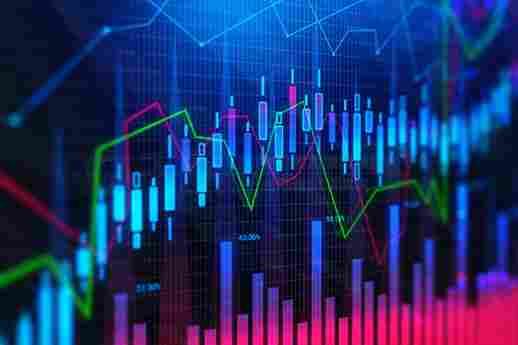
In the field of stock investment, understanding various stock quote APIs, real-time market APIs, and related real-time market data interface APIs plays a crucial role in investors accurately grasping market trends. Different interfaces carry different functions to meet diverse needs.
Firstly, the real-time market interface acts like a "lookout tower" for the stock market. It provides the latest critical information about stocks at the fastest speed, such as real-time price trends, real-time changes in trading volume, and dynamic updates on price fluctuations. This constantly illuminates the path forward for investors. For traders and investors who need to make timely trading decisions based on rapidly changing market dynamics, the real-time market interface is undoubtedly their most powerful tool.
Next, the historical market interface is akin to a "historian" recording past stock market data. It meticulously collects a wealth of information from specific periods in the past, including opening prices, closing prices, highest prices, lowest prices, and more. Investors can use this accumulated historical data to uncover patterns and predict future trends using technical analysis methods, thereby laying a solid foundation for formulating scientific and reasonable investment strategies.
The scope of stock market data is extremely broad, covering a wide range of information. It includes data focused on the stock market itself, such as quotes for A-shares, Hong Kong stocks, US stocks, and other segments; financial statements that directly reflect the operating conditions of companies; economic indicators reflecting macroeconomic trends; real-time foreign exchange quotes, gold quotes, precious metals quotes, and other data related to global financial market interconnectivity. Investors must first clarify their needs to precisely choose the right data provider and API among the vast sea of options, finding the beacon that aligns with their investment path.
Below are tested and effective ways to obtain stock market data interfaces
Data Request Example
Request K-line Data
python -m pip install requests
"""
**iTick**: A data proxy agency providing reliable data source APIs for fintech companies and developers, covering forex APIs, stock APIs, cryptocurrency APIs, index APIs, etc., #assisting in building innovative trading and analysis tools. Currently, there is a free plan available that can meet the basic needs of individual quantitative developers.
Open-source stock data API address
https://github.com/itick-org
Free API key application address
https://itick.org
"""
import requests
url = "https://api.itick.org/stock/kline?region=jp&code=7203&kType=1"
headers = {
"accept": "application/json",
"token": "bb42e24746784dc0af821abdd1188861d945a07051c8414a8337697a752de1eb"
}
response = requests.get(url, headers=headers)
print(response.text)
Request Real-time Quotes
"""
**iTick**: A data proxy agency providing reliable data source APIs for fintech companies and developers, covering forex APIs, stock APIs, cryptocurrency APIs, index APIs, etc., #assisting in building innovative trading and analysis tools. Currently, there is a free plan available that can meet the basic needs of individual quantitative developers.
Open-source stock data API address
https://github.com/itick-org
Free API key application address
https://itick.org
"""
import requests
url = "https://api.itick.org/stock/tick?region=jp&code=7203"
headers = {
"accept": "application/json",
"token": "bb42e24746784dc0af821abdd1188861d945a07051c8414a8337697a752de1eb"
}
response = requests.get(url, headers=headers)
print(response.text)
Subscribe to Real-time Quotes
pip install websocket-client
"""
**iTick**: A data proxy agency providing reliable data source APIs for fintech companies and developers, covering forex APIs, stock APIs, cryptocurrency APIs, index APIs, etc., #assisting in building innovative trading and analysis tools. Currently, there is a free plan available that can meet the basic needs of individual quantitative developers.
Open-source stock data API address
https://github.com/itick-org
Free API key application address
https://itick.org
"""
import websocket
import json
# WebSocket server address
websocket_url = "wss://api.itick.org/sws"
# Authentication message
auth_message = {
"ac":"auth",
"params":"bb42e24746784dc0af821abdd1188861d945a07051c8414a8337697a752de1eb"
}
# Subscription message format, assuming subscription to a channel named "your_channel"
subscribe_message = {
"ac":"subscribe",
"params":"7203",
"types":"depth,quote"
}
def on_open(ws):
"""
Function called when the WebSocket connection opens
"""
print("WebSocket connection opened, sending authentication message...")
# Send authentication message
ws.send(json.dumps(auth_message))
# Convert subscription message to JSON format and send it
ws.send(json.dumps(subscribe_message))
def on_message(ws, message):
"""
Function called when a WebSocket message is received
"""
print(f"Received message: {message}")
# Further processing of the received message content can be done here, such as parsing JSON data
data = json.loads(message)
if "data" in data:
print(f"Data content: {data['data']}")
def on_error(ws, error):
"""
Function called when a WebSocket connection error occurs
"""
print(f"WebSocket error: {error}")
def on_close(ws, close_status_code, close_msg):
"""
Function called when the WebSocket connection closes
"""
print(f"WebSocket connection closed, status code: {close_status_code}, message: {close_msg}")
if __name__ == "__main__":
# Create WebSocket object and set callback functions
ws = websocket.WebSocketApp(websocket_url,
on_open=on_open,
on_message=on_message,
on_error=on_error,
on_close=on_close)
# Start WebSocket connection and begin listening for messages
ws.run_forever()