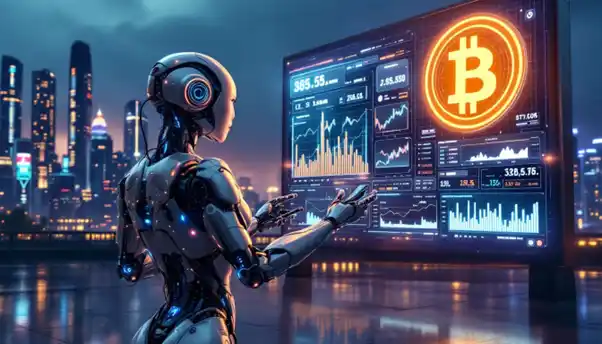
在量化交易领域,iTick 报价 API凭借其强大的多市场覆盖能力,已成为专业交易员的首选数据解决方案。其外汇 API支持全球主要货币对(如 EURUSD、GBPUSD)的毫秒级行情推送,包含 Bid/Ask 深度报价和实时波动率数据;股票 API则覆盖 A 股、港股及美股市场,提供 Level-2 逐笔成交和十档盘口信息。通过统一的 RESTful 接口,开发者可轻松获取标准化的 OHLCV 数据,实现外汇、股票等多资产策略的无缝适配。凭借高频低延迟特性,iTick API 特别适合日内交易策略开发,其历史数据回溯功能支持长达 15 年的日线级数据下载,为策略回测提供可靠支撑。
一、策略原理
双均线策略通过长短周期均线的交叉判断趋势方向:
- 金叉信号:短期均线上穿长期均线 → 做多
- 死叉信号:短期均线下穿长期均线 → 做空
- 趋势过滤:结合成交量或波动率指标增强信号有效性
二、数据准备
使用 iTick 报价源获取多市场数据:
"""
**iTick**:是一家数据代理机构,为金融科技公司和开发者提供可靠的数据源APIs,涵盖外汇API、股票API、加密货币API、指数API等,帮助构建创新的交易和分析工具,目前有免费的套餐可以使用基本可以满足个人量化开发者需求
https://github.com/itick-org
https://itick.org
"""
pip install itrade # iTick数据接口
数据获取示例(以 EURUSD 外汇对和贵州茅台股票为例):
from itrade import quote
# 获取外汇历史数据
eurusd_df = quote.get_kline(
symbol="EURUSD",
start_date="2023-01-01",
interval="15min",
market="forex"
)
# 获取股票历史数据
茅台_df = quote.get_kline(
symbol="600519.SH",
start_date="2023-01-01",
interval="30min",
market="stock"
)
三、策略实现
1. 双均线计算模块
import talib
def calculate_ma(df, short_window=20, long_window=60):
# 计算简单移动平均线
df['MA_SHORT'] = talib.SMA(df['close'], short_window)
df['MA_LONG'] = talib.SMA(df['close'], long_window)
# 计算交叉信号
df['cross_long'] = df['MA_SHORT'] > df['MA_LONG']
df['cross_short'] = df['MA_SHORT'] < df['MA_LONG']
# 生成交易信号
df['signal'] = 0
df.loc[df['cross_long'] & df['cross_long'].shift(1).eq(False), 'signal'] = 1 # 金叉
df.loc[df['cross_short'] & df['cross_short'].shift(1).eq(False), 'signal'] = -1 # 死叉
return df
2. 多市场交易逻辑
def execute_strategy(df, symbol, account_balance=100000):
# 初始化持仓与资金
position = 0
equity = account_balance
# 遍历交易信号
for i in range(1, len(df)):
current_signal = df['signal'].iloc[i]
prev_signal = df['signal'].iloc[i-1]
if current_signal == 1 and prev_signal != 1:
# 开多仓(外汇使用保证金交易)
if symbol.startswith("EURUSD"):
position = 1 # 1标准手
equity -= df['close'].iloc[i] * 100000 # 假设1标准手保证金
else:
# 股票交易(1手=100股)
shares = int(equity * 0.9 / df['close'].iloc[i]) // 100 * 100
position = shares
equity -= shares * df['close'].iloc[i]
elif current_signal == -1 and prev_signal != -1:
# 开空仓(股票需支持融券)
if symbol.startswith("600519"):
shares = int(equity * 0.9 / df['close'].iloc[i]) // 100 * 100
position = -shares
equity += shares * df['close'].iloc[i] # 融券卖出获得资金
# 平仓逻辑(此处简化处理,实际需考虑手续费)
if prev_signal != current_signal and position != 0:
equity += position * df['close'].iloc[i]
position = 0
return equity
四、策略回测
1. 多品种回测框架
def backtest_multiple_symbols(symbols):
results = {}
for symbol in symbols:
# 获取数据并处理
df = quote.get_kline(symbol, start_date="2023-01-01", interval="30min")
df = calculate_ma(df)
# 执行回测
final_equity = execute_strategy(df, symbol)
returns = (final_equity - 100000) / 100000 * 100
results[symbol] = {
"final_equity": final_equity,
"returns": returns
}
return results
2. 回测结果示例
symbols = ["EURUSD", "600519.SH", "XAUUSD"]
results = backtest_multiple_symbols(symbols)
print("策略回测结果:")
for symbol, res in results.items():
print(f"{symbol}: 最终权益{res['final_equity']:.2f}元,收益率{res['returns']:.2f}%")
五、策略优化方向
- 参数优化:使用遗传算法搜索最优均线组合
- 多资产配置:外汇 + 股票 + 商品组合降低风险
- 动态仓位管理:基于 ATR 调整头寸规模
- 机器学习增强:加入成交量加权均线等特征
六、iTick API 优势
- 多市场统一接口:外汇、股票、期货使用相同数据格式
- 高频数据支持:提供毫秒级 Tick 数据与历史 K 线
- 实时行情推送:WebSocket 接口实现低延迟数据更新
- 模拟交易环境:支持实盘 API 与回测框架无缝切换
七、注意事项
- 外汇交易需注意杠杆风险(通常 1:100~1:500)
- 股票融券交易受标的池限制
- 建议使用 iTick 的模拟交易接口进行策略验证
- 需根据市场特性调整滑点和手续费模型
通过本文的代码框架,可快速构建基于双均线的多市场量化策略。实际部署时建议结合 iTick 的实时数据流与交易网关,实现策略的自动化执行与风险监控。