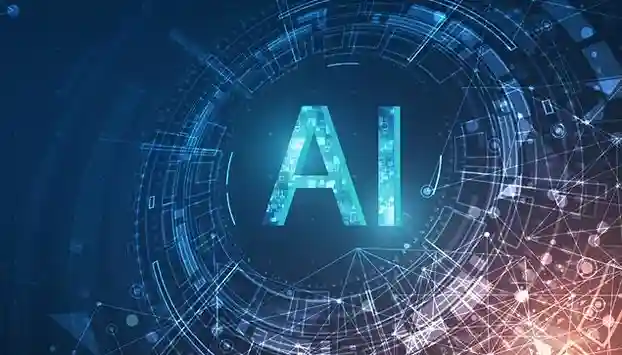
iTick 提供了强大的外汇报价 API、股票报价 API 和指数报价 API 服务,为量化策略的开发提供了丰富的数据支持。本文将详细介绍如何使用 Python 结合 EMA12 指标和 iTick 的报价 API 来构建一个简单的量化交易策略,并对该策略进行回测。
1. 引言
在量化交易领域,技术指标是构建交易策略的重要基础。iTick 提供了强大的外汇报价 API、股票报价 API 和指数报价 API 服务,为量化策略的开发提供了丰富的数据支持。本文将详细介绍如何使用 Python 结合 EMA12 指标和 iTick 的报价 API 来构建一个简单的量化交易策略,并对该策略进行回测。指数移动平均线(Exponential Moving Average,EMA)是一种广泛应用的技术指标,它能够更及时地反映价格的最新变化趋势。EMA12 即 12 周期的指数移动平均线,常被用于短期趋势的判断。
2. 准备工作
2.1 安装必要的库
首先,确保你已经安装了以下 Python 库:
pip install requests pandas numpy matplotlib
2.2 获取 iTick API 密钥
你需要在 iTick 平台注册账号并获取 API 密钥,以便能够调用其报价 API。
2.3 导入必要的库
import requests
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
3. 使用 iTick API 获取数据
3.1 定义 API 请求函数
def get_quote_data(symbol, api_key, start_date, end_date, interval):
url = f"https://api.itick.com/quote?symbol={symbol}&api_key={api_key}&start_date={start_date}&end_date={end_date}&interval={interval}"
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
df = pd.DataFrame(data['data'])
df['timestamp'] = pd.to_datetime(df['timestamp'])
df.set_index('timestamp', inplace=True)
return df
except requests.RequestException as e:
print(f"请求出错: {e}")
return None
except KeyError as e:
print(f"数据解析出错: {e}")
return None
3.2 获取数据示例
api_key = "your_api_key"
symbol = "EURUSD" # 外汇交易对,也可以替换为股票代码或指数代码
start_date = "2023-01-01"
end_date = "2023-12-31"
interval = "1d" # 数据间隔,这里设置为日线数据
data = get_quote_data(symbol, api_key, start_date, end_date, interval)
if data is not None:
print(data.head())
4. 计算 EMA12 指标
4.1 定义计算 EMA12 的函数
def calculate_ema12(data):
data['ema12'] = data['close'].ewm(span=12, adjust=False).mean()
return data
4.2 计算 EMA12 并展示结果
data = calculate_ema12(data)
if data is not None:
print(data[['close', 'ema12']].head())
5. 构建交易策略
5.1 定义交易信号生成函数
def generate_signals(data):
data['signal'] = 0
data.loc[data['close'] > data['ema12'], 'signal'] = 1 # 买入信号
data.loc[data['close'] < data['ema12'], 'signal'] = -1 # 卖出信号
data['position'] = data['signal'].diff()
return data
5.2 生成交易信号并展示结果
data = generate_signals(data)
if data is not None:
print(data[['close', 'ema12', 'signal', 'position']].head())
6. 策略回测
6.1 定义回测函数
def backtest(data):
initial_capital = float(100000.0)
positions = pd.DataFrame(index=data.index).fillna(0.0)
positions[symbol] = 100 * data['signal'] # 假设每次交易 100 单位
portfolio = positions.multiply(data['close'], axis=0)
pos_diff = positions.diff()
portfolio['holdings'] = (positions.multiply(data['close'], axis=0)).sum(axis=1)
portfolio['cash'] = initial_capital - (pos_diff.multiply(data['close'], axis=0)).sum(axis=1).cumsum()
portfolio['total'] = portfolio['cash'] + portfolio['holdings']
portfolio['returns'] = portfolio['total'].pct_change()
return portfolio
6.2 进行回测并展示结果
portfolio = backtest(data)
if portfolio is not None:
print(portfolio[['holdings', 'cash', 'total', 'returns']].head())
7. 策略可视化
7.1 绘制价格和 EMA12 曲线
if data is not None:
plt.figure(figsize=(12, 6))
plt.plot(data['close'], label='Close Price')
plt.plot(data['ema12'], label='EMA12')
plt.title(f'{symbol} Close Price and EMA12')
plt.xlabel('Date')
plt.ylabel('Price')
plt.legend()
plt.show()
7.2 绘制策略净值曲线
if portfolio is not None:
plt.figure(figsize=(12, 6))
plt.plot(portfolio['total'], label='Portfolio Value')
plt.title('Portfolio Value over Time')
plt.xlabel('Date')
plt.ylabel('Value')
plt.legend()
plt.show()
8. 总结
本文详细介绍了如何使用 Python 结合 EMA12 指标和 iTick 的外汇报价 API、股票报价 API、指数报价 API 服务来构建一个简单的量化交易策略,并对该策略进行了回测和可视化。通过以上步骤,你可以根据不同的交易品种和数据间隔,灵活调整策略参数,进一步优化策略。
需要注意的是,实际的量化交易还需要考虑交易成本、滑点、风险控制等因素,本文仅为一个基础的示例,旨在帮助你入门量化策略的开发和回测。在实际应用中,你可以结合更多的技术指标和交易规则,构建更加复杂和有效的量化交易策略。