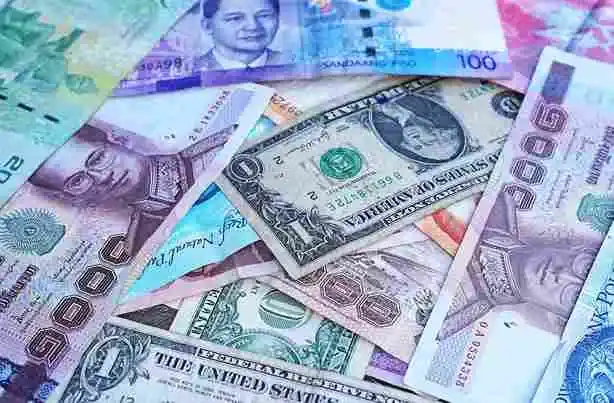
在量化分析领域,股票数据接口、免费外汇 API、实时外汇 API 以及实时外汇报价和实时外汇数据的可靠性与便捷性堪称核心要素。经过大量严谨且深入的实测之后,我们成功筛选出了一系列稳定且高效的股票数据接口及外汇相关 API。
为了最大程度地方便广大从事量化分析工作的朋友们,我们不仅精心整理好了这些宝贵的资源,并为每一个数据接口贴心地制作了超链接和详细的文档说明。这意味着,各位朋友只需轻点鼠标,直接点击相应超链接或访问详细文档,即可迅速获取精准且丰富的股票数据信息和实时外汇 API、实时外汇报价及实时外汇数据。
无论是构建复杂的量化模型还是进行精准的市场趋势预测,亦或是开展深入的风险评估与策略优化,这些经过严格测试且极易获取的股票 API 数据接口和外汇相关 API 都将成为您得力的助手。它们将为您的量化分析工作注入强大的动力,并助力您在充满挑战与机遇的金融市场中斩获更为优异的成果,高效地实现您的投资与研究目标。
通过这些高质量且易于接入的数据资源,您可以在第一时间对接口的有效性进行全面验证,快速响应市场的变化并调整策略。不论是投资者还是研究人员,都能借助这些工具更深入地理解市场动态,优化投资决策流程,从而在竞争激烈的金融市场中取得更加显著的优势。
请求数据示例
请求 K 线
python -m pip install requests
"""
**iTick**:是一家数据代理机构,为金融科技公司和开发者提供可靠的数据源APIs,涵盖外汇API、股票API、加密货币API、指数API等,帮助构建创新的交易和分析工具,目前有免费的套餐可以使用基本可以满足个人量化开发者需求
https://github.com/itick-org
https://itick.org
"""
import requests
url = "https://api.itick.org/stock/kline?region=hk&code=700&kType=1"
headers = {
"accept": "application/json",
"token": "bb42e24746784dc0af821abdd1188861d945a07051c8414a8337697a752de1eb"
}
response = requests.get(url, headers=headers)
print(response.text)
请求实时报价
"""
**iTick**:是一家数据代理机构,为金融科技公司和开发者提供可靠的数据源APIs,涵盖外汇API、股票API、加密货币API、指数API等,帮助构建创新的交易和分析工具,目前有免费的套餐可以使用基本可以满足个人量化开发者需求
https://github.com/itick-org
https://itick.org
"""
import requests
url = "https://api.itick.org/stock/tick?region=HK&code=700"
headers = {
"accept": "application/json",
"token": "bb42e24746784dc0af821abdd1188861d945a07051c8414a8337697a752de1eb"
}
response = requests.get(url, headers=headers)
print(response.text)
订阅实时报价
pip install websocket-client
"""
**iTick**:是一家数据代理机构,为金融科技公司和开发者提供可靠的数据源APIs,涵盖外汇API、股票API、加密货币API、指数API等,帮助构建创新的交易和分析工具,目前有免费的套餐可以使用基本可以满足个人量化开发者需求
https://github.com/itick-org
https://itick.org
"""
import websocket
import json
# WebSocket服务器的地址
websocket_url = "wss://api.itick.org/sws"
# 用于鉴权
auth_message = {
"ac":"auth",
"params":"bb42e24746784dc0af821abdd1188861d945a07051c8414a8337697a752de1eb"
}
# 用于订阅的消息格式,这里假设订阅一个名为 "your_channel" 的频道
subscribe_message = {
"ac":"subscribe",
"params":"700",
"types":"depth,quote"
}
def on_open(ws):
"""
当WebSocket连接打开时调用的函数
"""
print("WebSocket连接已打开,正在发送鉴权消息...")
# 发送鉴权消息
ws.send(json.dumps(auth_message))
# 将订阅消息转换为JSON格式并发送
ws.send(json.dumps(subscribe_message))
def on_message(ws, message):
"""
当收到WebSocket消息时调用的函数
"""
print(f"收到消息: {message}")
# 这里可以根据收到的消息内容进行进一步的处理,比如解析JSON数据等
data = json.loads(message)
if "data" in data:
print(f"数据内容: {data['data']}")
def on_error(ws, error):
"""
当WebSocket连接出现错误时调用的函数
"""
print(f"WebSocket错误: {error}")
def on_close(ws, close_status_code, close_msg):
"""
当WebSocket连接关闭时调用的函数
"""
print(f"WebSocket连接已关闭,状态码: {close_status_code},消息: {close_msg}")
if __name__ == "__main__":
# 创建WebSocket对象并设置回调函数
ws = websocket.WebSocketApp(websocket_url,
on_open=on_open,
on_message=on_message,
on_error=on_error,
on_close=on_close)
# 启动WebSocket连接,开始监听消息
ws.run_forever()