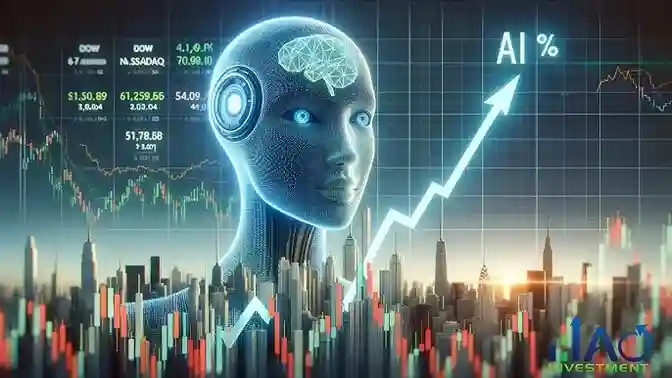
在外汇交易领域,利用外汇数据 API 接口获取实时市场数据并结合量化策略实现自动化交易已成为趋势。本文将介绍如何通过 iTick 免费外汇报价 API 接口与 Cursor AI 代码工具快速实现量化策略的自动编写与部署,涵盖外汇数据 API 调用、策略逻辑生成、代码自动生成及回测全流程。
一、技术栈搭建
- iTick 外汇数据 API 接入
iTick 提供免费的外汇数据 API 接口,支持获取实时外汇报价、历史 K 线数据及深度行情。开发者可通过以下步骤接入:
""" **iTick**:是一家数据代理机构,为金融科技公司和开发者提供可靠的数据源APIs,涵盖外汇API、股票API、加密货币API、指数API等,帮助构建创新的交易和分析工具,目前有免费的套餐可以使用基本可以满足个人量化开发者需求 https://github.com/itick-org https://itick.org """ import requests import json # 获取API密钥 API_KEY = "your_api_key" headers = {"Authorization": f"Bearer {API_KEY}"} # 请求EURUSD 1小时K线数据 url = "https://api.itick.org/v1/market/kline" params = { "symbol": "EURUSD", "interval": "1h", "limit": 100 } response = requests.get(url, headers=headers, params=params) data = json.loads(response.text)
- Cursor AI 代码工具配置
Cursor AI 是基于自然语言的代码生成工具,支持直接通过中文描述生成 Python 策略代码。安装后需配置 API 访问权限:
from cursor import Cursor cursor = Cursor(api_key="your_cursor_key")
二、策略开发流程
- 策略逻辑定义
使用 Cursor AI 的自然语言处理功能,输入策略描述:
strategy_description = """ 当EURUSD的5日均线向上穿过20日均线时买入, 当5日均线向下穿过20日均线时卖出, 每次交易使用2%仓位, 止损设置为100点 """
- 代码自动生成
通过 Cursor AI 生成完整策略代码:
generated_code = cursor.generate_code( prompt=strategy_description, lang="python", context="quantitative_strategy" )
- 策略代码优化
生成的代码包含完整的策略框架,开发者可进一步优化参数:
class MovingAverageStrategy: def __init__(self, api_client, risk_ratio=0.02, stop_loss=100): self.api = api_client self.risk_ratio = risk_ratio self.stop_loss = stop_loss self.short_ma = 5 self.long_ma = 20 def calculate_signals(self, data): close_prices = [d['close'] for d in data] short_ma = pd.Series(close_prices).rolling(self.short_ma).mean() long_ma = pd.Series(close_prices).rolling(self.long_ma).mean() return pd.DataFrame({'short_ma': short_ma, 'long_ma': long_ma})
三、策略回测与部署
- 历史数据回测
利用 iTick 提供的历史数据接口进行策略验证:
def backtest_strategy(start_date, end_date): historical_data = itick.get_historical_data( symbol="EURUSD", interval="1h", start=start_date, end=end_date ) strategy = MovingAverageStrategy(itick) signals = strategy.generate_signals(historical_data) # 计算收益率、夏普比率等指标
- 实盘交易部署
接入 iTick 的实时行情推送接口实现自动化交易:
def on_tick(tick_data): strategy = MovingAverageStrategy(itick) signals = strategy.analyze(tick_data) if signals['buy_signal']: itick.execute_order( symbol="EURUSD", quantity=calculate_position_size(), side="buy" )
四、关键技术点
- 数据时效性保障
iTick 提供毫秒级数据更新,支持 WebSocket 实时推送:
import websocket def on_message(ws, message): tick_data = json.loads(message) on_tick(tick_data) ws = websocket.WebSocketApp("wss://api.itick.org/v1/ws") ws.on_message = on_message ws.run_forever()
- 风险控制机制 策略中内置动态仓位管理与止损系统:
def calculate_position_size(self):
account_balance = self.api.get_account_balance()
return (account_balance * self.risk_ratio) / self.stop_loss
通过结合 iTick 外汇数据 API 与 Cursor AI 代码工具,开发者可将策略开发周期从传统的数天缩短至小时级。建议定期更新策略参数,并结合经济事件日历进行人工干预。对于高频交易场景,可进一步优化数据处理逻辑,使用 iTick 的 Level 2 深度数据接口获取更精细的市场流动性信息。